Unity Software is, like, the OG game engine, right? Seriously, it’s everywhere. From indie darlings to AAA titles, you’ll find Unity powering a huge chunk of the games you play. This isn’t just about making games though; it’s a whole ecosystem, with tools, assets, and a massive community backing it all up. We’re diving deep into what makes Unity tick, from its core features to advanced techniques.
We’ll explore everything from setting up your first scene to mastering complex shaders. We’ll cover the Asset Store, different rendering pipelines (URP, HDRP – what even
-are* those?), and even touch on mobile and VR development. Think of this as your ultimate guide to conquering the world of Unity game development. Get ready to level up your skills!
Unity Software’s Core Features
Unity is a wildly popular game engine used by indie developers and AAA studios alike. Its strength lies in its accessibility, robust feature set, and large community support. This makes it a great choice for everything from simple 2D mobile games to complex VR experiences. We’ll dive into some of its core functionalities and compare it to a major competitor.
Key Features of the Unity Game Engine
Unity boasts a comprehensive suite of tools for game development. Its core features include a powerful scene editor for visually constructing game worlds, a versatile animation system for creating realistic and expressive characters, and a robust physics engine that simulates realistic interactions between objects. Beyond this, it offers built-in support for 2D and 3D game development, a comprehensive asset store with pre-made assets, and a vast array of plugins to extend functionality.
The engine also supports various platforms, allowing developers to easily deploy their games to PC, mobile, consoles, and web browsers. This cross-platform compatibility is a huge advantage, saving developers significant time and effort.
Comparison of Unity and Unreal Engine Features
While both Unity and Unreal Engine are leading game engines, they cater to slightly different needs. Unity is generally considered more beginner-friendly due to its simpler interface and easier learning curve. Unreal Engine, on the other hand, is known for its incredibly powerful rendering capabilities and is often preferred for high-fidelity graphics. Unity’s asset store provides a massive library of pre-made assets, making it easier to quickly prototype and develop games, while Unreal Engine’s Blueprint visual scripting system offers a less code-intensive approach to development.
Ultimately, the “better” engine depends on the specific project requirements and the developer’s experience level.
Unity’s Scripting Capabilities Using C#
Unity primarily uses C# for scripting, offering a powerful and versatile language for controlling game logic and behavior. C#’s object-oriented nature allows for well-structured and maintainable code, making it ideal for large and complex projects. Developers can use C# to create custom game mechanics, manage player input, implement AI, and control virtually every aspect of the game. The integration between C# and Unity’s editor is seamless, allowing for rapid iteration and testing of code changes.
For example, a simple script to move a game object could be written in just a few lines of C#. This ease of use combined with the power of C# is a major reason for Unity’s popularity.
Designing a Simple 2D Game Using Core Unity Features
Let’s design a basic 2D platformer. This example leverages only core Unity features, avoiding external assets or advanced techniques.
Step | Description | Unity Action | Code Example (C#) |
---|---|---|---|
1 | Create the Player | Create a Sprite object and assign a sprite image. Add a Rigidbody2D component for physics. | //No code needed for this step |
2 | Implement Movement | Add a C# script to control horizontal movement using Input.GetAxis(“Horizontal”). | using UnityEngine;public class PlayerMovement : MonoBehaviour public float speed = 5f; Rigidbody2D rb; void Start() rb = GetComponent |
3 | Add Jumping | Add a jump function to the script, triggered by pressing the spacebar. Use rb.AddForce(). | void Update() if (Input.GetButtonDown("Jump")) rb.AddForce(new Vector2(0f, jumpForce), ForceMode2D.Impulse); public float jumpForce = 10f; |
4 | Create Platforms | Create Sprite objects for platforms, and position them in the scene. Add Box Collider 2D components. | //No code needed for this step |
Unity’s Asset Store Ecosystem
The Unity Asset Store is a massive online marketplace brimming with pre-made assets, tools, and resources designed to accelerate game development. Think of it as a one-stop shop for everything from stunning 3D models and realistic sound effects to complex AI systems and powerful plugins.
It’s a vital part of the Unity ecosystem, offering developers a shortcut to building richer, more polished games without starting from scratch.The Asset Store provides a huge range of benefits for developers of all skill levels, but it’s not without its potential drawbacks. Understanding both the advantages and disadvantages is crucial for making informed decisions about asset integration.
Five Essential Assets on the Unity Asset Store
Choosing just five “essential” assets is tricky, as it depends heavily on project needs. However, these five categories represent assets frequently used and highly valued by many Unity developers:
- High-Quality Character Models: Ready-to-animate characters save immense time and effort in modeling. Look for models with good topology and UV mapping for optimal performance and ease of texturing.
- Realistic Sound Effects: A well-crafted soundscape significantly enhances immersion. Packs offering a variety of ambient sounds, weapon effects, and character voices are invaluable.
- Particle Effects: From simple sparks to elaborate explosions, particle systems add visual flair. Asset packs provide pre-made effects, customizable to suit various game scenarios.
- Post-Processing Effects: Enhance the visual quality of your game with realistic lighting, bloom, and depth of field effects. These assets often include easy-to-use controls for fine-tuning the look.
- UI Toolkit: A well-designed user interface is crucial for any game. Assets providing customizable UI elements, pre-made menus, and efficient UI frameworks streamline the process.
Benefits and Drawbacks of Using Asset Store Assets
The Asset Store offers significant advantages, primarily saving developers considerable time and resources. However, relying solely on pre-made assets can lead to unforeseen challenges.
- Benefits: Faster prototyping, access to professional-quality assets, cost-effectiveness (compared to in-house creation), expanded skillset through learning from existing assets.
- Drawbacks: Potential for asset incompatibility, licensing restrictions (consider carefully the terms of use), dependence on external developers for updates and support, potential for lower-quality assets if not vetted properly.
Examples of Successful Games Using Primarily Asset Store Assets, Unity software
While it’s difficult to definitively say a game wasprimarily* built using Asset Store assets (developers rarely publicize this level of detail), many indie games leverage the store extensively. Games that frequently utilize Asset Store assets often have a faster development cycle and lower initial development costs. The success hinges on smart selection, skillful integration, and strong game design. Imagine a small team using pre-made character models, environmental assets, and UI elements to build a charming 2D platformer, focusing their energies on gameplay and narrative rather than spending months on asset creation.
Comparison of Three Character Animation Asset Packs
This table compares three hypothetical character animation asset packs, highlighting key features and price points. Remember that actual asset pack details will vary.
Asset Pack Name | Animation Styles | Number of Animations | Price (USD) |
---|---|---|---|
AnimPack Pro | Realistic, stylized, cartoon | 50+ | $99 |
CharacterMotion Essentials | Realistic, stylized | 30 | $49 |
CartoonAnimator Lite | Cartoon | 15 | $29 |
Game Development Workflow in Unity
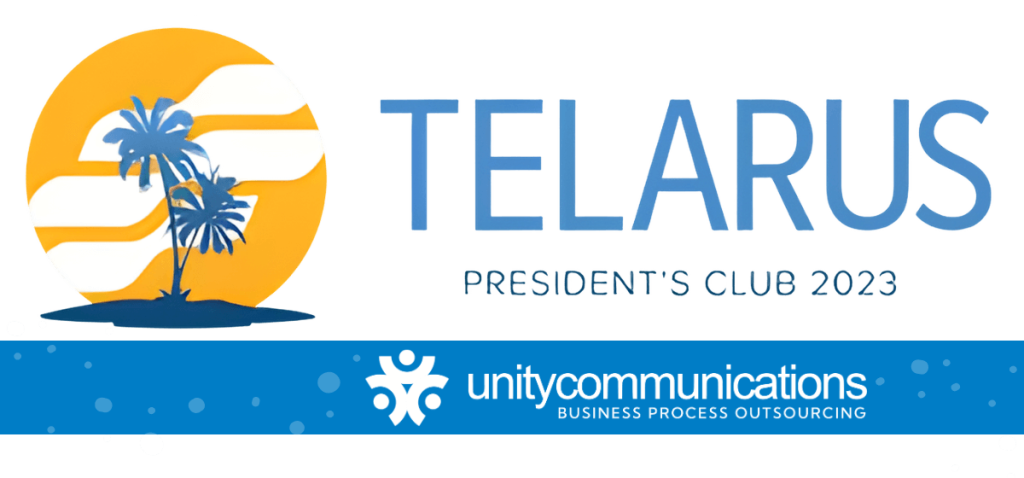
So, you’ve got Unity installed, maybe even dabbled with a few tutorials. Now it’s time to dive into the actual process of building a 3D game. It’s a journey, not a sprint, but with a solid workflow, you can keep your project organized and your sanity intact. Think of it like building a house – you wouldn’t just start slapping bricks together, right?
You’d need blueprints, a plan, and a team (even if that team is just you!).The typical workflow for creating a 3D game in Unity involves several iterative stages, often overlapping and refining as the project progresses. It’s a cyclical process of planning, prototyping, building, testing, and refining, constantly evaluating and iterating. From initial concept to polished product, the process requires careful organization and attention to detail.
Version Control in Unity Projects
Using version control, specifically Git, is absolutely crucial for any Unity project, regardless of size or scope. Imagine accidentally deleting a week’s worth of work – a nightmare scenario easily avoided with a good version control system. Git allows you to track changes, revert to previous versions, collaborate with others seamlessly, and generally maintain a clean and organized project history.
Popular platforms like GitHub, GitLab, and Bitbucket offer repositories to store your project and its version history. Think of it as a safety net and a collaborative tool, allowing for multiple developers to work concurrently without overwriting each other’s changes. Branching allows for experimental features without affecting the main codebase, ensuring a stable development process.
Importing and Using External 3D Models
Bringing in pre-made assets can dramatically speed up development. Unity supports a wide range of 3D model formats, including FBX, OBJ, and 3DS. The import process is usually straightforward: you simply drag and drop the model file into the Project window in Unity. Unity will then automatically generate a preview and allow you to adjust import settings, such as scaling, texture import options, and mesh optimization settings.
Poorly optimized models can severely impact performance, so understanding these settings is vital. For example, you might reduce the polygon count of a high-resolution model for mobile platforms to improve frame rate without significant visual loss. After importing, the model becomes an asset available for placement and manipulation within your scenes.
Setting Up a Basic Scene in Unity
Creating a basic scene is your first step into the world of Unity game development. This simple, step-by-step guide will help you get started.
- Create a New Project: Launch Unity and create a new 3D project. Choose a location to save your project files.
- Navigate the Interface: Familiarize yourself with the main windows: the Hierarchy (objects in your scene), the Project (assets), the Inspector (properties of selected objects), the Scene (the 3D view), and the Game (preview window).
- Add a Light Source: Navigate to GameObject > Light > Directional Light. This will illuminate your scene.
- Import a 3D Model (Optional): If you have a 3D model, import it into the project (as described in the previous section). Drag and drop the model from the Project window into the Scene view.
- Add a Camera: Create a Main Camera by navigating to GameObject > Create Empty and renaming it “Main Camera.” Add a Camera component to this object via the Inspector.
- Position the Camera: Adjust the camera’s position and rotation in the Scene view to frame your model (or the empty scene) appropriately. This allows you to view the scene from a desired perspective.
- Save the Scene: Save your scene by clicking File > Save Scene. Give it a descriptive name, such as “MyFirstScene”.
Unity’s Rendering Pipeline: Unity Software
Unity offers several rendering pipelines, each catering to different project needs and performance targets. Choosing the right pipeline significantly impacts your game’s visual fidelity and performance. Understanding their strengths and weaknesses is crucial for optimizing your development process.
Available Rendering Pipelines
Unity provides three main rendering pipelines: Built-in Render Pipeline (BRP), Universal Render Pipeline (URP), and High-Definition Render Pipeline (HDRP). BRP is the legacy pipeline, while URP and HDRP are more modern and flexible options. BRP is generally simpler but less efficient and feature-rich than the others. URP is a lightweight and versatile pipeline suitable for a wide range of projects, while HDRP is designed for high-fidelity visuals and complex scenes, often used in AAA titles.
Performance Characteristics Comparison
The performance of each pipeline varies greatly depending on the scene complexity and target platform. BRP generally offers the simplest performance profile, but this comes at the cost of features and potential optimizations. URP strikes a balance between performance and visual fidelity, making it suitable for mobile and mid-range hardware. HDRP, however, demands significantly more processing power, making it best suited for high-end PCs and consoles where visual quality is prioritized.
Frame rates can be significantly lower with HDRP in resource-intensive scenes compared to URP or BRP on the same hardware. The overhead associated with HDRP’s advanced features like screen-space reflections and global illumination contributes to this performance difference. Specific performance benchmarks would vary depending on hardware, scene complexity, and rendering settings.
HDRP Advantages and Disadvantages
HDRP’s primary advantage lies in its ability to render highly realistic visuals. It supports advanced features like physically based rendering (PBR), high dynamic range (HDR), advanced lighting models, and sophisticated post-processing effects. This allows developers to create stunningly realistic scenes with detailed lighting, shadows, and reflections. However, HDRP’s complexity comes at a cost. It requires more powerful hardware, increases development complexity, and can lead to longer build times and higher memory consumption.
The steep learning curve associated with its advanced features can also be a barrier for some developers. A project requiring photorealistic visuals would benefit from HDRP’s capabilities, but a mobile game with simpler visuals would likely be better served by URP.
Scene Demonstrating URP and HDRP Differences
Imagine a forest scene. In the URP version, the trees might have simpler textures and shaders, the lighting might be less nuanced, and reflections might be less detailed or entirely absent. Shadows might be less soft and realistic. Overall, the scene would have a slightly cartoonish or stylized look, suitable for a mobile game or a less graphically intensive title.In contrast, the HDRP version of the same forest scene would boast highly detailed tree models with realistic bark textures and leaf shaders.
The lighting would be much more dynamic and realistic, with soft shadows and subtle ambient occlusion. Screen-space reflections would show detailed reflections of the trees and sky on surfaces like water or wet leaves. Global illumination would add realism to the lighting by simulating indirect lighting effects. The overall visual quality would be significantly higher, resulting in a photorealistic experience.
The difference in visual fidelity would be immediately apparent, with HDRP offering a significantly more immersive and realistic representation of the forest environment.
Unity’s Physics Engine
Unity’s physics engine is a powerful tool that simulates realistic physical interactions within your game world. It uses a sophisticated system to calculate forces, collisions, and movements of objects, making your games feel more dynamic and engaging. Understanding how this engine works is key to creating believable and fun gameplay.
At its core, Unity’s physics engine uses a physics simulation based on Newtonian physics. It calculates the forces acting on objects (gravity, friction, applied forces) and updates their positions and velocities accordingly. This happens in discrete time steps, meaning the engine calculates the physics state at regular intervals. The smaller the time step, the more accurate (and computationally expensive) the simulation becomes.
The engine also handles collision detection, determining when objects come into contact and calculating the resulting forces and impulses. This is done using various collision detection algorithms to optimize performance. Complex shapes are often approximated by simpler shapes to speed up calculations.
Collider Types
Colliders are essential components that define the shape and physical properties of a GameObject for physics interactions. Choosing the right collider type is crucial for performance and accuracy. Incorrect collider selection can lead to unexpected behavior like objects passing through each other or experiencing unrealistic bouncing.
- Box Collider: A simple, rectangular collider, ideal for basic shapes and often the most efficient.
- Sphere Collider: A spherical collider, perfect for balls, planets, or any round object. Generally more efficient than a mesh collider for round shapes.
- Capsule Collider: A cylinder with hemispherical caps at each end. Useful for characters or objects with a cylindrical form.
- Mesh Collider: Uses the actual geometry of a 3D model. Offers the most accurate collision detection but can be computationally expensive, especially with complex meshes. Often used as a last resort.
- Wheel Collider: Specialized collider for simulating wheels, providing features like wheel slip and suspension.
Implementing Realistic Physics
Creating realistic physics involves careful consideration of several factors. Accurate mass values, appropriate friction coefficients, and correctly configured colliders are crucial. For instance, a heavier object should fall faster than a lighter one, and a rough surface should produce more friction than a smooth one. Experimentation and iterative adjustments are essential to achieve the desired level of realism.
Consider a simple example of a ball bouncing on the ground. You would assign a Sphere Collider to the ball GameObject, set its mass, and adjust the bounciness (a property of the physics material) to control how much energy is lost on each bounce. Similarly, for a car, you might use Wheel Colliders and adjust parameters like suspension stiffness and friction to simulate realistic driving behavior.
The more you refine these parameters, the more lifelike your game will feel.
Rigidbodies and Joints
Rigidbodies are components that enable physics simulation for GameObjects. They allow objects to be affected by gravity, collisions, and forces. Joints connect rigidbodies, creating constraints that simulate hinges, springs, or other physical connections. For example, a hinge joint could be used to model a door, while a spring joint could simulate a suspension system. Properly configuring mass, drag, angular drag, and other Rigidbody properties is essential for achieving desired movement and interactions.
Mobile Game Development with Unity
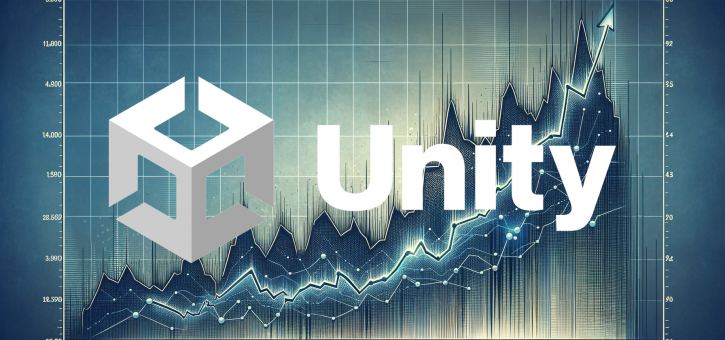
Unity’s versatility extends seamlessly to mobile game development, offering a powerful platform for creating engaging experiences across iOS and Android devices. However, this convenience comes with its own set of challenges, primarily centered around performance optimization and platform-specific considerations. Successfully navigating these hurdles is key to delivering a polished and enjoyable mobile gaming experience.
Mobile Game Development Challenges and Considerations
Developing for mobile presents unique obstacles compared to desktop or console development. Limited processing power, memory constraints, and diverse device hardware configurations necessitate careful planning and optimization. Battery life is another critical factor; lengthy gameplay sessions should not drain a user’s phone rapidly. Furthermore, understanding the nuances of different mobile operating systems (iOS and Android) and their respective app stores is crucial for successful deployment and marketing.
Different screen sizes and resolutions require adaptable UI designs, and network connectivity issues must be accounted for to ensure a smooth user experience, even in areas with limited internet access. Finally, mobile app stores have specific guidelines and submission processes that must be adhered to.
Optimizing Game Performance on Mobile Devices
Optimizing a Unity mobile game involves a multi-pronged approach. Reducing the polygon count of 3D models, using lower-resolution textures, and employing level of detail (LOD) techniques significantly impact performance. Minimizing draw calls by batching similar objects together is also vital. Efficient scripting practices, such as avoiding unnecessary calculations and using object pooling to reuse game objects, are crucial.
Profiling tools within Unity allow developers to pinpoint performance bottlenecks and make targeted optimizations. For example, the Unity Profiler helps identify areas of the code that are consuming the most CPU or GPU resources. By addressing these performance bottlenecks, developers can create a smooth and responsive gaming experience even on lower-end devices. A game that runs smoothly on a range of devices will have a larger potential audience.
Integrating In-App Purchases into a Unity Mobile Game
In-app purchases (IAPs) provide a monetization strategy for mobile games. Unity provides robust tools for integrating IAPs through its IAP system or third-party plugins. The process generally involves setting up a store connection (e.g., connecting to Google Play Billing or Apple’s App Store), defining consumable and non-consumable in-game items, and implementing the purchase flow within the game. Careful consideration must be given to the design of IAPs to avoid creating a pay-to-win experience that alienates players.
A well-integrated IAP system offers optional enhancements without compromising the core gameplay experience, ensuring a positive user experience. For instance, offering cosmetic items or time-saving boosts rather than directly impacting gameplay balance can encourage purchases without disrupting fairness.
Building and Deploying a Simple Mobile Game to an Android Device
Building and deploying a simple Unity mobile game to Android involves several steps. First, the game project must be configured for Android in Unity’s build settings, selecting the appropriate Android SDK and other necessary components. Then, the game is built within the Unity editor, generating an Android APK (Android Package Kit) file. This APK file can then be installed on an Android device either via USB connection or by uploading to platforms like Google Play Console.
Before deploying to a wider audience, thorough testing on a variety of Android devices is crucial to ensure compatibility and performance across different hardware configurations. This process involves testing on devices with varying screen sizes, processors, and amounts of RAM to identify and resolve any issues that may arise. This testing phase is vital for delivering a high-quality user experience.
Unity’s Animation System
Unity’s animation system is a powerful toolset for bringing your game characters and objects to life. It allows you to create everything from subtle movements to complex, dynamic animations, significantly enhancing the overall visual appeal and immersion of your game. The system relies heavily on animation clips and animation controllers, which work together to manage and play animations efficiently.The core components of Unity’s animation system are animation clips and animation controllers.
Animation clips contain the actual animation data, recording the position, rotation, and scale of objects over time. These clips can be created in external 3D modeling software like Blender or Maya and imported into Unity, or they can be created directly within the Unity editor using tools like the Animation window. Animation controllers, on the other hand, act as managers, determining which animation clip to play at any given time, often based on game events or character states.
This allows for dynamic and responsive animations.
Skeletal Animation and Animation Blending
Skeletal animation is the most common animation technique used in Unity. It involves creating a skeleton or rig for your 3D model, which consists of a hierarchy of bones connected by joints. The animation data then manipulates the positions and rotations of these bones, causing the model to deform and move realistically. Think of it like the bones and joints in a human body.
Moving one bone affects the position of others connected to it.Animation blending allows you to smoothly transition between different animation clips. This is crucial for creating realistic and natural movements. For example, you might blend between a walking animation and a running animation to create a smooth acceleration. Different blending methods exist, such as linear blending and crossfading, each offering varying degrees of smoothness and control.
The choice of method depends on the desired effect and the complexity of the animation. For instance, a quick transition between idle and attack might use linear blending, while a character smoothly changing from a walk to a run benefits from crossfading.
Creating Realistic Character Animations
Creating realistic character animations requires careful planning and execution. It starts with a well-designed 3D model that is appropriately rigged for animation. The quality of the animation is directly tied to the quality of the model and the rigging. Next, you need to capture the nuances of movement. This involves considering factors such as weight transfer, foot placement, and the overall timing and pacing of the animation.
Advanced techniques, like root motion (controlling the character’s root bone to move the entire character) and inverse kinematics (IK), can help achieve even more realistic movements. For instance, accurately animating a character picking up an object requires attention to hand and finger movements, as well as the object’s physics and interaction with the hand.
Rigging a Simple 3D Model for Animation
Before you can animate a 3D model in Unity, it needs to be rigged. Rigging involves creating a skeleton and attaching it to the model’s mesh. Here’s a breakdown of the process:
The rigging process ensures the 3D model is prepared for animation. A well-executed rig is crucial for smooth and natural-looking animations. Improper rigging can lead to distorted or unnatural movements. The choice of rigging method depends on the model’s complexity and the desired level of animation control.
- Import the 3D Model: Import your 3D model (in a format like FBX or OBJ) into Unity.
- Create a Skeleton: Use a 3D modeling software (like Blender or Maya) to create a skeleton for your model. This involves creating bones and connecting them to form a hierarchy that mirrors the model’s structure. The root bone is usually placed at the center of the model’s base.
- Weight Painting: Assign weights to the vertices of the model’s mesh, defining how each vertex is influenced by the bones. This step ensures that the mesh deforms correctly when the bones move.
- Import the Rigged Model: Import the rigged model (including the skeleton and weights) back into Unity.
- Test the Rig: In Unity, test the rig by manually moving the bones to check for any deformities or issues in the mesh deformation.
Unity and Virtual Reality (VR)
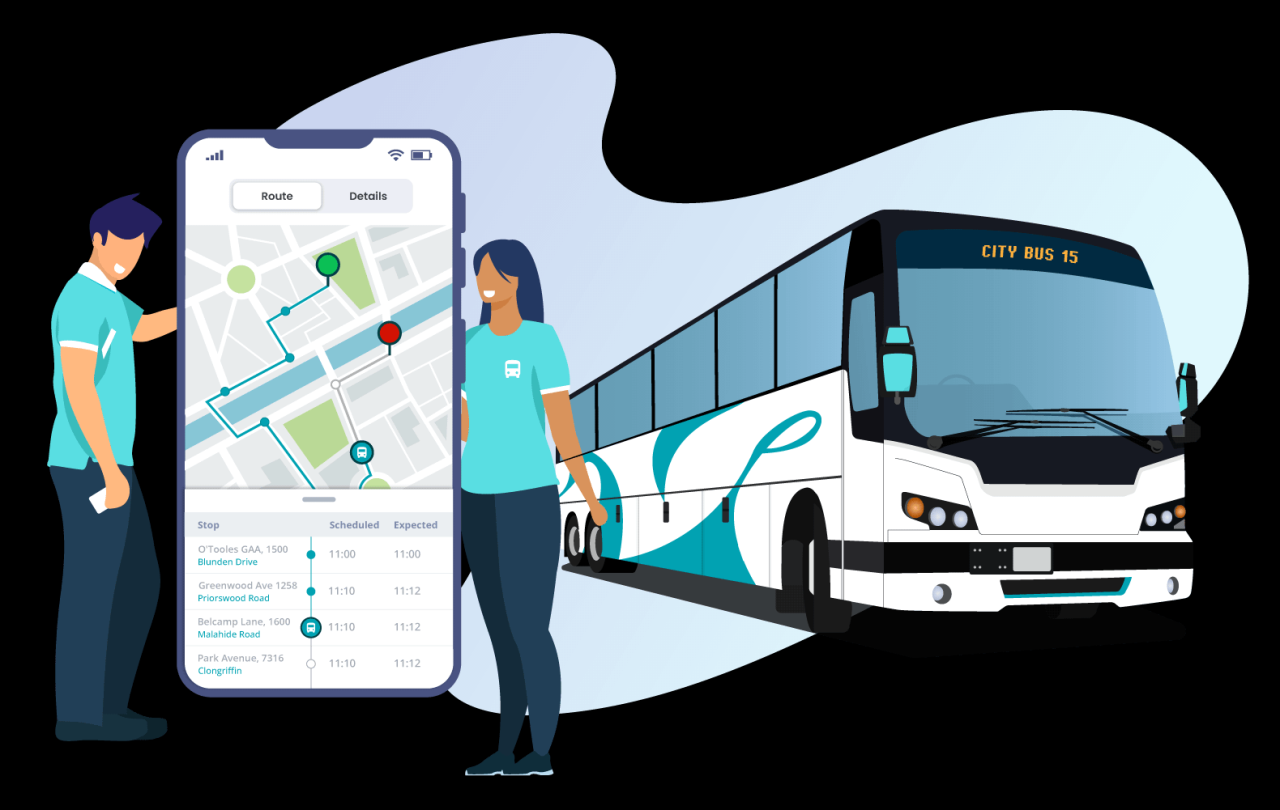
Unity has become a dominant force in VR development, offering a robust and versatile platform for creating immersive experiences. Its ease of use, combined with powerful features and a vast asset store, makes it an attractive choice for both indie developers and large studios tackling the challenges of VR. This section explores Unity’s capabilities in VR development, the hurdles faced by VR developers, and showcases successful examples.Unity’s support for VR development is extensive, encompassing various VR platforms like Oculus Rift, HTC Vive, Windows Mixed Reality, and standalone headsets like Oculus Quest.
It provides built-in tools and packages to simplify the process of creating VR applications, including features for handling VR input, managing head tracking, and optimizing performance for VR headsets. The platform’s adaptability allows developers to target a wide range of devices with relative ease, maximizing potential reach.
VR Development Challenges
Developing compelling VR experiences presents unique challenges beyond traditional game development. One major hurdle is motion sickness, often caused by discrepancies between what the user sees and feels. Careful consideration of camera movement, teleportation systems, and smooth transitions are crucial to mitigate this issue. Another challenge lies in designing intuitive and engaging interactions within the virtual environment.
VR controllers require innovative interaction designs, often deviating from traditional keyboard and mouse schemes. Optimization for VR hardware is also paramount, as VR applications are often more demanding on system resources than traditional games, demanding careful asset management and performance optimization strategies. Finally, the design of compelling VR experiences requires careful consideration of the user’s physical space and comfort.
Successful VR Games Built with Unity
Several successful VR games leverage Unity’s capabilities. Beat Saber*, a rhythm game requiring precise movements, demonstrates the power of Unity’s physics engine and VR interaction capabilities. The game’s intuitive gameplay and vibrant visuals have made it a major success story in the VR gaming landscape.
Half-Life
Alyx*, a critically acclaimed VR title, showcases Unity’s ability to deliver high-fidelity visuals and immersive storytelling within a VR environment. Its intricate level design and realistic interactions highlight the potential of VR gaming. These examples underscore Unity’s role in pushing the boundaries of VR gaming.
Designing a Basic VR Interaction System
A basic VR interaction system could be built around a raycast system. The user’s VR controller emits a ray that detects interactable objects in the scene. When the ray intersects with an object, the object can be highlighted visually. A trigger button press could then initiate an interaction, such as picking up the object. The object’s position and rotation could be updated relative to the controller’s position and orientation, allowing the user to manipulate it.
This simple system provides a foundation for more complex interactions, such as object manipulation, menu navigation, and environmental interaction. More advanced systems might incorporate hand tracking for more natural interactions, or haptic feedback for increased immersion.
Unity’s Networking Capabilities
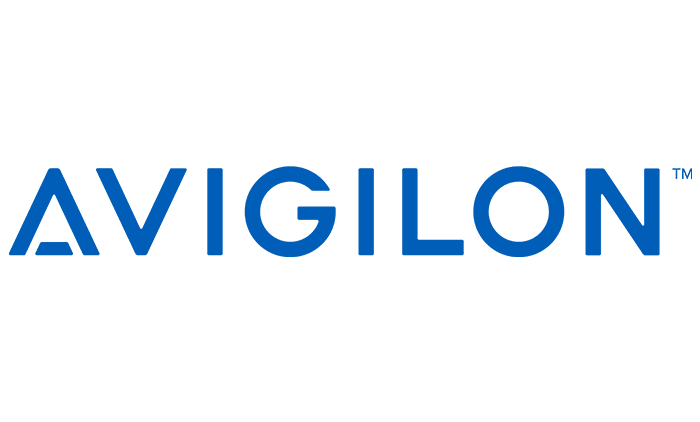
Unity offers robust networking features that allow developers to create engaging multiplayer experiences, ranging from simple cooperative games to complex massively multiplayer online (MMO) titles. These features abstract away much of the low-level networking complexity, enabling developers to focus on game logic and design. Understanding these capabilities is crucial for building successful online games.Unity provides several networking solutions, each with its strengths and weaknesses.
Choosing the right solution depends on factors like the game’s scale, complexity, and target platform.
Unity’s Built-in Networking (UNET)
UNET, while now considered legacy, was Unity’s original built-in networking solution. It provided a relatively straightforward API for creating multiplayer games. However, it lacked features found in newer solutions and has limited scalability for larger player counts. It’s still usable for smaller projects or as a learning tool to understand fundamental networking concepts. A simple example of using UNET might involve two players controlling characters that can see and interact with each other in the same virtual world, each client sending updates on their character’s position and actions to the server which then relays the information to other clients.
Mirror
Mirror is a popular open-source networking solution built on top of Unity’s low-level networking features. It offers a more modern and flexible approach than UNET, supporting various networking models (client-server, peer-to-peer, etc.). Mirror is known for its performance and scalability, making it suitable for a wider range of projects. Its modular design allows developers to customize its behavior to suit their specific needs.
A key advantage of Mirror is its active community support and frequent updates.
Photon
Photon is a commercial networking solution that provides a comprehensive suite of tools and features for building multiplayer games. It’s a highly scalable solution often used in large-scale MMOs. Photon’s ease of use and robust features make it a popular choice for developers who prioritize ease of development over complete control. Photon’s cloud services handle much of the server infrastructure, reducing the burden on developers.
Implementing Multiplayer Features: A Simple Example
Let’s consider a simple example: a top-down shooter where players can shoot projectiles at each other. Using Mirror, each player’s client would handle local input (e.g., aiming and shooting). When a player fires a shot, the client sends the projectile’s initial position, velocity, and other relevant data to the server. The server then validates the data (to prevent cheating) and broadcasts the information to all other clients.
Each client would then locally render the projectile’s movement, ensuring consistent gameplay across all players. This approach minimizes latency and prevents client-side prediction from causing inconsistencies.
Designing a Simple Online Multiplayer Game
To design a simple online multiplayer game in Unity, we’d follow these steps:
- Game Concept and Design: Define the core gameplay loop, player interactions, and overall game mechanics. For example, a simple cooperative game where players work together to defeat waves of enemies.
- Networking Solution Selection: Choose a networking solution (Mirror, Photon, or another) based on project requirements and scalability needs. For a small-scale game, Mirror might be a good choice due to its open-source nature and flexibility.
- Server Architecture: Decide on the server architecture (client-server or peer-to-peer). Client-server is generally preferred for larger games due to its better control and scalability.
- Data Synchronization: Determine how game state will be synchronized between clients and the server. Techniques like interpolation and extrapolation can be used to smooth out network latency.
- Input Handling: Design a system for handling player input and ensuring that actions are properly replicated across all clients. Server-side validation is crucial to prevent cheating.
- Game Logic Implementation: Implement the core game logic, ensuring that it works correctly in a multiplayer environment.
- Testing and Iteration: Thoroughly test the game with multiple players to identify and fix any bugs or performance issues.
Unity’s User Interface (UI) System
Unity’s UI system provides a flexible and powerful way to create interactive interfaces for your games. It’s built using a canvas-based system, allowing for easy scaling and resolution independence across various devices. Understanding its core components and best practices is crucial for designing engaging and user-friendly game experiences.Unity’s UI system utilizes a hierarchy of elements, similar to the game object hierarchy.
The core component is the Canvas, which acts as a container for all UI elements. Within the Canvas, you’ll find various UI elements such as buttons, text fields, images, sliders, and more. Each element has properties to control its appearance, behavior, and interaction. These properties can be adjusted in the Inspector panel, allowing for precise customization.
The system also supports anchoring and constraints, enabling responsive layouts that adapt to different screen sizes and resolutions.
UI Element Creation and Interaction
Creating interactive UI elements involves attaching scripts to these elements and utilizing Unity’s event system. For example, to create a clickable button, you would add a Button component to a UI Image. Then, you’d write a script that listens for the OnClick event, triggering a specific function when the button is pressed. This function could then manage game logic, such as starting a new level or opening a menu.
Similarly, you can handle input events for other UI elements like text fields (for user input) and sliders (for adjusting game settings). The event system acts as a central hub for managing all user interactions with the UI.
Best Practices for User-Friendly UI Design
Effective UI design is crucial for a positive user experience. Key considerations include clear visual hierarchy, intuitive navigation, and consistent design language. A well-structured UI guides users effortlessly through the game’s functionalities. For instance, using contrasting colors to highlight important buttons or employing visual cues like arrows to indicate interactive elements greatly improves usability. Maintaining consistent button styles, font sizes, and spacing throughout the UI creates a cohesive and professional look.
Testing your UI on various devices and screen sizes is essential to ensure responsiveness and accessibility. Finally, consider using established UI design principles, such as Gestalt principles, to create a visually appealing and intuitive layout.
Simple Menu System Design
Let’s design a simple pause menu. Imagine a rectangular menu that appears centrally on the screen when the game is paused. The menu would contain three buttons: “Resume Game,” “Main Menu,” and “Options.”The visual layout would be as follows: A dark translucent background covers the game screen. Centered within this background is a clean, rectangular panel with rounded corners.
So, Unity software’s pretty awesome for game dev, right? But sometimes you need to share those killer game design docs, and that’s where grabbing a copy of Adobe Acrobat comes in handy – you can easily download it from here: adobe acrobat download. Then, you can efficiently manage and share your polished PDFs, ensuring everyone’s on the same page for that next Unity project milestone.
The panel houses three buttons arranged vertically, each with clear, concise text labels. “Resume Game” sits at the top, followed by “Main Menu” in the middle, and “Options” at the bottom. Each button would have a consistent size, spacing, and visual style, maintaining visual harmony. A simple, visually appealing font would be used for all text, ensuring readability. The entire menu design would be visually uncluttered and easy to navigate, prioritizing user experience.
The buttons would utilize the OnClick event to trigger corresponding game functions.
Advanced Techniques in Unity
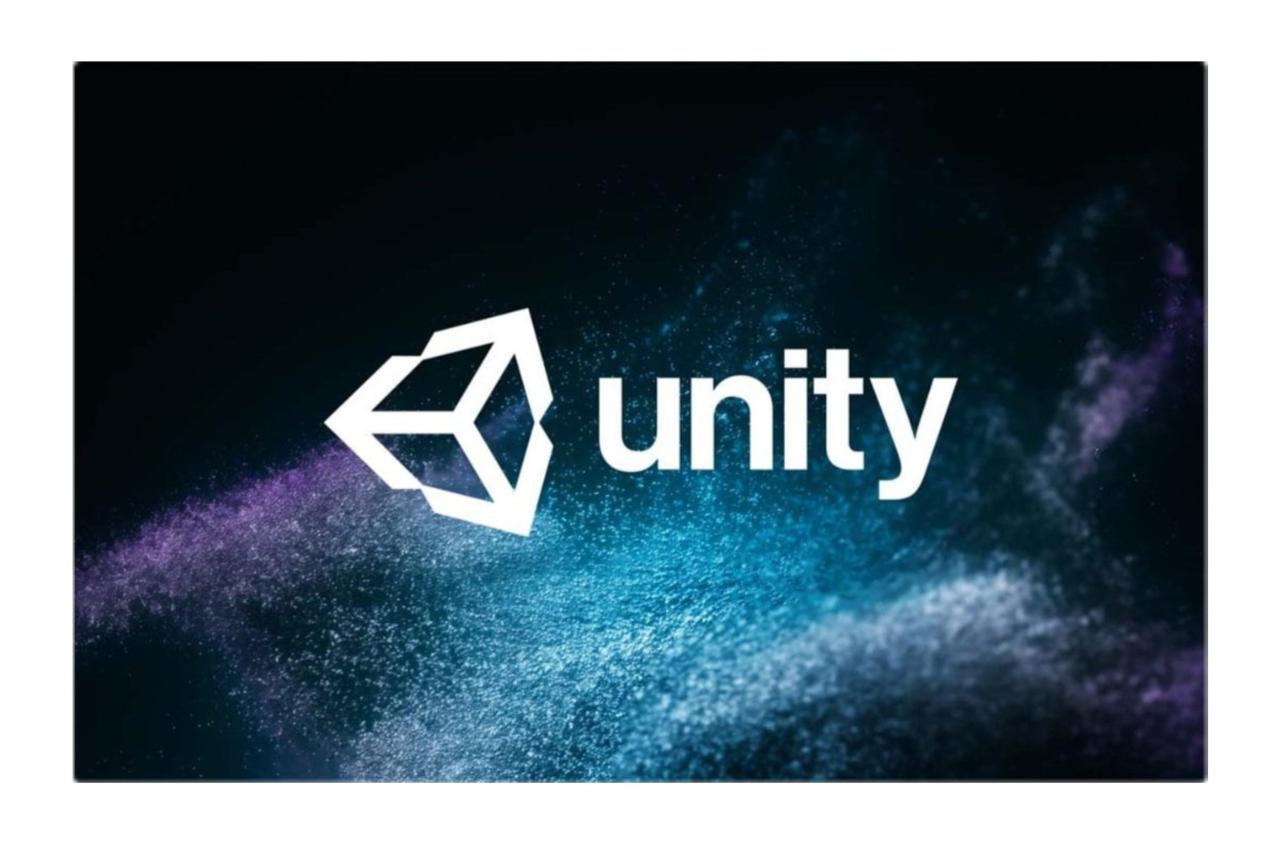
Unity’s power extends far beyond the basics. Mastering advanced techniques unlocks the potential to create truly stunning and performant games. This section dives into shader programming, compute shaders, procedural generation, and raycasting—essential skills for any Unity developer aiming to build high-quality experiences.
Advanced Shader Programming in Unity
Shader programming allows for precise control over how objects are rendered. Instead of relying on Unity’s built-in shaders, developers can create custom shaders using the Shader Graph visual editor or by writing code in HLSL (High-Level Shading Language). This opens doors to creating unique visual effects, stylized rendering, and optimizing performance for specific hardware. For example, a custom shader could implement a cel-shaded effect, mimicking the look of hand-drawn animation, or create a realistic water shader with dynamic reflections and refractions.
Advanced techniques include using surface shaders for efficient material rendering and utilizing vertex and fragment shaders for fine-grained control over the rendering pipeline. Understanding concepts like texture sampling, lighting calculations, and shader s is crucial for effective shader development.
Compute Shaders for Performance Optimization
Compute shaders are powerful tools for offloading computationally intensive tasks from the main thread to the GPU. This parallel processing capability significantly boosts performance, especially in scenarios involving large-scale computations like physics simulations, pathfinding, or procedural generation. Instead of performing these calculations on the CPU, which can lead to frame rate drops, compute shaders leverage the GPU’s parallel processing power to execute them concurrently, resulting in smoother gameplay.
For instance, a compute shader could efficiently simulate fluid dynamics, generate a large terrain, or process vast amounts of particle data. The key is to identify computationally expensive tasks and strategically utilize compute shaders to optimize performance.
Procedural Generation Techniques in Unity
Procedural generation automates the creation of game content, such as terrains, levels, or even entire worlds. This technique allows for the generation of vast and varied content without manual creation, leading to increased replayability and reduced development time. Common algorithms used include Perlin noise for generating natural-looking textures and terrains, L-systems for creating branching structures like trees, and cellular automata for simulating emergent behavior.
For example, a game could procedurally generate a unique dungeon layout each time the player starts a new game, ensuring a fresh experience with each playthrough. Implementing these techniques requires understanding algorithms and data structures to efficiently generate and manage large amounts of game data.
Raycasting for Game Interactions
Raycasting is a technique used to detect collisions between a ray (a line) and objects in the scene. It’s fundamental for implementing interactions like picking objects with the mouse, detecting line-of-sight, or creating projectile-based gameplay. Unity provides built-in raycasting functionality through the `Physics.Raycast` function, which returns information about the first object the ray collides with, such as the point of impact and the object itself.
For example, a first-person shooter game might use raycasting to detect when the player’s weapon hits an enemy, while a strategy game could use it to select units based on mouse clicks. The ability to efficiently perform raycasting is crucial for creating responsive and interactive game experiences. Advanced techniques involve using layers to control which objects raycasts interact with and optimizing raycasting performance for large scenes.
Final Review
So, there you have it – a whirlwind tour through the amazing world of Unity software! From basic scene setup to advanced shader programming, we’ve covered a lot of ground. Remember, the key to mastering Unity is practice and exploration. Don’t be afraid to experiment, break things (and then fix them!), and most importantly, have fun! The possibilities are endless, so get out there and build something awesome.
Helpful Answers
Is Unity free?
There’s a free version with limitations, but the Pro version unlocks more features for larger projects.
What programming languages does Unity support?
C# is the primary language, but you can also use others through plugins.
How steep is the learning curve?
It depends on your prior experience. The basics are pretty accessible, but mastering advanced features takes time and effort.
Is Unity good for 2D games?
Absolutely! Unity’s equally adept at 2D and 3D game development.
What’s the best way to learn Unity?
A mix of tutorials, documentation, and hands-on projects is ideal. The Unity Learn platform is a great resource.